A lot of beginner tutorials start with “Hello World” examples. There are plenty of websites that use a calculator application as a kind of “Hello World” for GUI beginners. Calculators are a good way to learn because they have a set of widgets that you need to lay out in an orderly fashion. They also require a certain amount of logic to make them work correctly. For this calculator, let’s focus on being able to do the following:
- Addition
- Subtraction
- Multiplication
- Division
I think that supporting these four functions is a great starting place and also give you plenty of room for enhancing the application on your own.
Figuring Out the Logic
One of the first items that you will need to figure out is how to actually execute the equations that you build. For example, let’s say that you have the following equation:
1 + 2 * 5
What is the solution? If you read it left-to-right, the solution would seem to be 3 * 5 or 15. But multiplication has a higher precedence than addition, so it would actually be 10 + 1 or 11. How do you figure out precedence in code? You could spend a lot of time creating a string parser that groups numbers by the operand or you could use Python’s built-in `eval` function. The eval() function is short for evaluate and will evaluate a string as if it was Python code.
A lot of Python programmers actually discourage the user of eval(). Let’s find out why.
Is eval() Evil?
The eval() function has been called “evil” in the past because it allows you to run strings as code, which can open up your application’s to nefarious evil-doers. You have probably read about SQL injection where some websites don’t properly escape strings and accidentally allowed dishonest people to edit their database tables by running SQL commands via strings. The same concept can happen in Python when using the eval() function. A common example of how eval could be used for evil is as follows:
eval("__import__('os').remove('file')")
This code will import Python’s os module and call its remove() function, which would allow your users to delete files that you might not want them to delete. There are a couple of approaches for avoiding this issue:
- Don’t use eval()
- Control what characters are allowed to go to eval()
Since you will be creating the user interface for this application, you will also have complete control over how the user enters characters. This actually can protect you from eval’s insidiousness in a straight-forward manner. You will learn two methods of using wxPython to control what gets passed to eval(), and then you will learn how to create a custom eval() function at the end of the article.
Designing the Calculator
Let’s take a moment and try to design a calculator using the constraints mentioned at the beginning of the chapter. Here is the sketch I came up with:
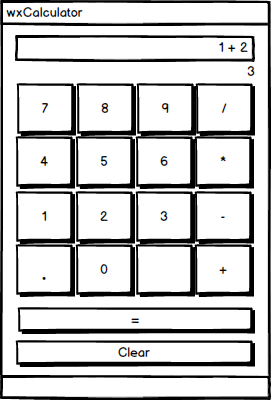
Note that you only care about basic arithmetic here. You won’t have to create a scientific calculator, although that might be a fun enhancement to challenge yourself with. Instead, you will create a nice, basic calculator.
Let’s get started!
Creating the Initial Calculator
Whenever you create a new application, you have to consider where the code will go. Does it go in the wx.Frame class, the wx.Panel class, some other class or what? It is almost always a mix of different classes when it comes to wxPython. As is the case with most wxPython applications, you will want to start by coming up with a name for your application. For simplicity’s sake, let’s call it wxcalculator.py for now.
The first step is to add some imports and subclass the Frame widget. Let’s take a look:
import wx class CalcFrame(wx.Frame): def __init__(self): super().__init__( None, title="wxCalculator", size=(350, 375)) panel = CalcPanel(self) self.SetSizeHints(350, 375, 350, 375) self.Show() if __name__ == '__main__': app = wx.App(False) frame = CalcFrame() app.MainLoop()
This code is very similar to what you have seen in the past. You subclass wx.Frame and give it a title and initial size. Then you instantiate the panel class, CalcPanel (not shown) and you call the SetSizeHints() method. This method takes the smallest (width, height) and the largest (width, height) that the frame is allowed to be. You may use this to control how much your frame can be resized or in this case, prevent any resizing. You can also modify the frame’s style flags in such a way that it cannot be resized too.
Here’s how:
class CalcFrame(wx.Frame): def __init__(self): no_resize = wx.DEFAULT_FRAME_STYLE & ~ (wx.RESIZE_BORDER | wx.MAXIMIZE_BOX) super().__init__( None, title="wxCalculator", size=(350, 375), style=no_resize) panel = CalcPanel(self) self.Show()
Take a look at the no_resize variable. It is creating a wx.DEFAULT_FRAME_STYLE and then using bitwise operators to remove the resizable border and the maximize button from the frame.
Let’s move on and create the CalcPanel:
class CalcPanel(wx.Panel): def __init__(self, parent): super().__init__(parent) self.last_button_pressed = None self.create_ui()
I mentioned this in an earlier chapter, but I think it bears repeating here. You don’t need to put all your interfacer creation code in the init method. This is an example of that concept. Here you instantiate the class, set the last_button_pressed attribute to None and then call create_ui(). That is all you need to do here.
Of course, that begs the question. What goes in the create_ui() method? Well, let’s find out!
def create_ui(self): main_sizer = wx.BoxSizer(wx.VERTICAL) font = wx.Font(12, wx.MODERN, wx.NORMAL, wx.NORMAL) self.solution = wx.TextCtrl(self, style=wx.TE_RIGHT) self.solution.SetFont(font) self.solution.Disable() main_sizer.Add(self.solution, 0, wx.EXPAND|wx.ALL, 5) self.running_total = wx.StaticText(self) main_sizer.Add(self.running_total, 0, wx.ALIGN_RIGHT) buttons = [['7', '8', '9', '/'], ['4', '5', '6', '*'], ['1', '2', '3', '-'], ['.', '0', '', '+']] for label_list in buttons: btn_sizer = wx.BoxSizer() for label in label_list: button = wx.Button(self, label=label) btn_sizer.Add(button, 1, wx.ALIGN_CENTER|wx.EXPAND, 0) button.Bind(wx.EVT_BUTTON, self.update_equation) main_sizer.Add(btn_sizer, 1, wx.ALIGN_CENTER|wx.EXPAND) equals_btn = wx.Button(self, label='=') equals_btn.Bind(wx.EVT_BUTTON, self.on_total) main_sizer.Add(equals_btn, 0, wx.EXPAND|wx.ALL, 3) clear_btn = wx.Button(self, label='Clear') clear_btn.Bind(wx.EVT_BUTTON, self.on_clear) main_sizer.Add(clear_btn, 0, wx.EXPAND|wx.ALL, 3) self.SetSizer(main_sizer)
This is a decent chunk of code, so let’s break it down a bit:
def create_ui(self): main_sizer = wx.BoxSizer(wx.VERTICAL) font = wx.Font(12, wx.MODERN, wx.NORMAL, wx.NORMAL)
Here you create the sizer that you will need to help organize the user interface. You will also create a wx.Font object, which is used to modifying the default font of widgets like wx.TextCtrl or wx.StaticText. This is helpful when you want a larger font size or a different font face for your widget than what comes as the default.
self.solution = wx.TextCtrl(self, style=wx.TE_RIGHT) self.solution.SetFont(font) self.solution.Disable() main_sizer.Add(self.solution, 0, wx.EXPAND|wx.ALL, 5)
These next three lines create the wx.TextCtrl, set it to right-justified (wx.TE_RIGHT), set the font and `Disable()` the widget. The reason that you want to disable the widget is because you don’t want the user to be able to type any string of text into the control.
As you may recall, you will be using eval() for evaluating the strings in that widget, so you can’t allow the user to abuse that. Instead, you want fine-grained control over what the user can enter into that widget.
self.running_total = wx.StaticText(self) main_sizer.Add(self.running_total, 0, wx.ALIGN_RIGHT)
Some calculator applications have a running total widget underneath the actual “display”. A simple way to add this widget is via the wx.StaticText widget.
Now let’s add main buttons you will need to use a calculator effectively:
buttons = [['7', '8', '9', '/'], ['4', '5', '6', '*'], ['1', '2', '3', '-'], ['.', '0', '', '+']] for label_list in buttons: btn_sizer = wx.BoxSizer() for label in label_list: button = wx.Button(self, label=label) btn_sizer.Add(button, 1, wx.ALIGN_CENTER|wx.EXPAND, 0) button.Bind(wx.EVT_BUTTON, self.update_equation) main_sizer.Add(btn_sizer, 1, wx.ALIGN_CENTER|wx.EXPAND)
Here you create a list of lists. In this data structure, you have the primary buttons used by your calculator. You will note that the there is a blank string in the last list that will be used to create a button that doesn’t do anything. This is to keep the layout correct. Theoretically, you could update this calculator down the road such that that button could be percentage or do some other function.
The next step is to create a the buttons, which you can do by looping over the list. Each nested list represents a row of buttons. So for each row of buttons, you will create a horizontally oriented wx.BoxSizer and then loop over the row of widgets to add them to that sizer. Once every button is added to the row sizer, you will add that sizer to your main sizer. Note that each of these button’s is bound to the `update_equation` event handler as well.
Now you need to add the equals button and the button that you may use to clear your calculator:
equals_btn = wx.Button(self, label='=') equals_btn.Bind(wx.EVT_BUTTON, self.on_total) main_sizer.Add(equals_btn, 0, wx.EXPAND|wx.ALL, 3) clear_btn = wx.Button(self, label='Clear') clear_btn.Bind(wx.EVT_BUTTON, self.on_clear) main_sizer.Add(clear_btn, 0, wx.EXPAND|wx.ALL, 3) self.SetSizer(main_sizer)
In this code snippet you create the “equals” button which you then bind to the on_total event handler method. You also create the “Clear” button, for clearing your calculator and starting over. The last line sets the panel’s sizer.
Let’s move on and learn what most of the buttons in your calculator are bound to:
def update_equation(self, event): operators = ['/', '*', '-', '+'] btn = event.GetEventObject() label = btn.GetLabel() current_equation = self.solution.GetValue() if label not in operators: if self.last_button_pressed in operators: self.solution.SetValue(current_equation + ' ' + label) else: self.solution.SetValue(current_equation + label) elif label in operators and current_equation is not '' \ and self.last_button_pressed not in operators: self.solution.SetValue(current_equation + ' ' + label) self.last_button_pressed = label for item in operators: if item in self.solution.GetValue(): self.update_solution() break
This is an example of binding multiple widgets to the same event handler. To get information about which widget has called the event handler, you can call the `event` object’s GetEventObject() method. This will return whatever widget it was that called the event handler. In this case, you know you called it with a wx.Button instance, so you know that wx.Button has a `GetLabel()` method which will return the label on the button. Then you get the current value of the solution text control.
Next you want to check if the button’s label is an operator (i.e. /, *, -, +). If it is, you will change the text controls value to whatever is currently in it plus the label. On the other hand, if the label is not an operator, then you want to put a space between whatever is currently in the text box and the new label. This is for presentation purposes. You could technically skip the string formatting if you wanted to.
The last step is to loop over the operands and check if any of them are currently in the equation string. If they are, then you will call the update_solution() method and break out of the loop.
Now you need to write the update_solution() method:
def update_solution(self): try: current_solution = str(eval(self.solution.GetValue())) self.running_total.SetLabel(current_solution) self.Layout() return current_solution except ZeroDivisionError: self.solution.SetValue('ZeroDivisionError') except: pass
Here is where the “evil” eval() makes its appearance. You will extract the current value of the equation from the text control and pass that string to eval(). Then convert that result back to a string so you can set the text control to the newly calculated solution. You want to wrap the whole thin in a try/except statement to catch errors, such as the ZeroDivisionError. The last except statement is known as a bare except and should really be avoided in most cases. For simplicity, I left it in there, but feel free to delete those last two lines if they offend you.
The next method you will want to take a look at is the on_clear() method:
def on_clear(self, event): self.solution.Clear() self.running_total.SetLabel('')
This code is pretty straight-forward. All you need to do is call your solution text control’s Clear() method to empty it out. You will also want to clear the `running_total` widget, which is an instance of wx.StaticText. That widget does not have a Clear() method, so instead you will call SetLabel() and pass in an empty string.
The last method you will need to create is the on_total() event handler, which will calculate the total and also clear out your running total widget:
def on_total(self, event): solution = self.update_solution() if solution: self.running_total.SetLabel('')
Here you can call the update_solution() method and get the result. Assuming that all went well, the solution will appear in the main text area and the running total will be emptied.
Here is what the calculator looks like when I ran it on a Mac:

And here is what the calculator looks like on Windows 10:
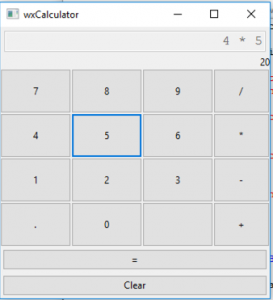
Let’s move on and learn how you might allow the user to use their keyboard in addition to your widgets to enter an equation.
Using Character Events
Most calculators will allow the user to use the keyboard when entering values. In this section, I will show you how to get started adding this ability to your code. The simplest method to use to make this work is to bind the wx.TextCtrl to the wx.EVT_TEXT event. I will be using this method for this example. However another way that you could do this would be to catch wx.EVT_KEY_DOWN and then analyze the key codes. That method is a bit more complex though.
The first item that we need to change is our CalcPanel‘s constructor:
# wxcalculator_key_events.py import wx class CalcPanel(wx.Panel): def __init__(self, parent): super().__init__(parent) self.last_button_pressed = None self.whitelist = ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '-', '+', '/', '*', '.'] self.on_key_called = False self.empty = True self.create_ui()
Here you add a whitelist attribute and a couple of simple flags, self.on_key_called and self.empty. The white list are the only characters that you will allow the user to type in your text control. You will learn about the flags when we actually get to the code that uses them.
But first, you will need to modify the create_ui() method of your panel class. For brevity, I will only reproduce the first few lines of this method:
def create_ui(self): main_sizer = wx.BoxSizer(wx.VERTICAL) font = wx.Font(12, wx.MODERN, wx.NORMAL, wx.NORMAL) self.solution = wx.TextCtrl(self, style=wx.TE_RIGHT) self.solution.SetFont(font) self.solution.Bind(wx.EVT_TEXT, self.on_key) main_sizer.Add(self.solution, 0, wx.EXPAND|wx.ALL, 5) self.running_total = wx.StaticText(self) main_sizer.Add(self.running_total, 0, wx.ALIGN_RIGHT)
Feel free to download the full source from Github or refer to the code in the previous section. The main differences here in regards to the text control is that you are no longer disabling it and you are binding it to an event: wx.EVT_TEXT.
Let’s go ahead an write the on_key() method:
def on_key(self, event): if self.on_key_called: self.on_key_called = False return key = event.GetString() self.on_key_called = True if key in self.whitelist: self.update_equation(key)
Here you check to see if the self.on_key_called flag is True. If it is, we set it back to False and `return` early. The reason for this is that when you use your mouse to click a button, it will cause EVT_TEXT to fire. The `update_equation()` method will get the contents of the text control which will be the key we just pressed and add the key back to itself, resulting in a double value. This is one way to workaround that issue.
You will also note that to get the key that was pressed, you can call the event object’s GetString() method. Then you will check to see if that key is in the white list. If it is, you will update the equation.
The next method you will need to update is update_equation():
def update_equation(self, text): operators = ['/', '*', '-', '+'] current_equation = self.solution.GetValue() if text not in operators: if self.last_button_pressed in operators: self.solution.SetValue(current_equation + ' ' + text) elif self.empty and current_equation: # The solution is not empty self.empty = False else: self.solution.SetValue(current_equation + text) elif text in operators and current_equation is not '' \ and self.last_button_pressed not in operators: self.solution.SetValue(current_equation + ' ' + text) self.last_button_pressed = text self.solution.SetInsertionPoint(-1) for item in operators: if item in self.solution.GetValue(): self.update_solution() break
Here you add a new elif that checks if the self.empty flag is set and if the current_equation has anything in it. In other words, if it is supposed to be empty and it’s not, then we set the flag to False because it’s not empty. This prevents a duplicate value when the keyboard key is pressed. So basically you need two flags to deal with duplicate values that can be caused because you decided to allow users to use their keyboard.
The other change to this method is to add a call to SetInsertionPoint() on your text control, which will put the insertion point at the end of the text control after each update.
The last required change to the panel class happens in the on_clear() method:
def on_clear(self, event): self.solution.Clear() self.running_total.SetLabel('') self.empty = True self.solution.SetFocus()
This change was done by adding two new lines to the end of the method. The first is to reset self.empty back to True. The second is to call the text control’s SetFocus() method so that the focus is reset to the text control after it has been cleared.
You could also add this SetFocus() call to the end of the on_calculate() and the on_total() methods. This should keep the text control in focus at all times. Feel free to play around with that on your own.
Creating a Better eval()
Now that you have looked at a couple of different methods of keeping the “evil” eval() under control, let’s take a few moments to learn how you can create a custom version of eval() on your own. Python comes with a couple of handy built-in modules called ast and operator. The ast module is an acronym that stands for “Abstract Syntax Trees” and is used “for processing trees of the Python abstract syntax grammar” according to the documentation. You can think of it as a data structure that is a representation of code. You can use the ast module to create a compiler in Python.
The operator module is a set of functions that correspond to Python’s operators. A good example would be operator.add(x, y) which is equivalent to the expression x+y. You can use this module along with the `ast` module to create a limited version of eval().
Let’s find out how:
import ast import operator allowed_operators = {ast.Add: operator.add, ast.Sub: operator.sub, ast.Mult: operator.mul, ast.Div: operator.truediv} def noeval(expression): if isinstance(expression, ast.Num): return expression.n elif isinstance(expression, ast.BinOp): print('Operator: {}'.format(expression.op)) print('Left operand: {}'.format(expression.left)) print('Right operand: {}'.format(expression.right)) op = allowed_operators.get(type(expression.op)) if op: return op(noeval(expression.left), noeval(expression.right)) else: print('This statement will be ignored') if __name__ == '__main__': print(ast.parse('1+4', mode='eval').body) print(noeval(ast.parse('1+4', mode='eval').body)) print(noeval(ast.parse('1**4', mode='eval').body)) print(noeval(ast.parse("__import__('os').remove('path/to/file')", mode='eval').body))
Here you create a dictionary of allowed operators. You map ast.Add to operator.add, etc. Then you create a function called `noeval` that accepts an `ast` object. If the expression is just a number, you return it. However if it is a BinOp instance, than you print out the pieces of the expression. A BinOp is made up of three parts:
- The left part of the expression
- The operator
- The right hand of the expression
What this code does when it finds a BinOp object is that it then attempts to get the type of ast operation. If it is one that is in our allowed_operators dictionary, then you call the mapped function with the left and right parts of the expression and return the result.
Finally if the expression is not a number or one of the approved operators, then you just ignore it. Try playing around with this example a bit with various strings and expressions to see how it works.
Once you are done playing with this example, let’s integrate it into your calculator code. For this version of the code, you can call the Python script wxcalculator_no_eval.py. The top part of your new file should look like this:
# wxcalculator_no_eval.py import ast import operator import wx class CalcPanel(wx.Panel): def __init__(self, parent): super().__init__(parent) self.last_button_pressed = None self.create_ui() self.allowed_operators = { ast.Add: operator.add, ast.Sub: operator.sub, ast.Mult: operator.mul, ast.Div: operator.truediv}
The main differences here is that you now have a couple of new imports (i.e. ast and operator) and you will need to add a Python dictionary called self.allowed_operators. Next you will want to create a new method called noeval():
def noeval(self, expression): if isinstance(expression, ast.Num): return expression.n elif isinstance(expression, ast.BinOp): return self.allowed_operators[ type(expression.op)](self.noeval(expression.left), self.noeval(expression.right)) return ''
This method is pretty much exactly the same as the function you created in the other script. It has been modified slightly to call the correct class methods and attributes however. The other change you will need to make is in the update_solution() method:
def update_solution(self): try: expression = ast.parse(self.solution.GetValue(), mode='eval').body current_solution = str(self.noeval(expression)) self.running_total.SetLabel(current_solution) self.Layout() return current_solution except ZeroDivisionError: self.solution.SetValue('ZeroDivisionError') except: pass
Now the calculator code will use your custom eval() method and keep you protected from the potentially harmfulness of eval(). The code that is in Github has the added protection of only allowing the user to use the onscreen UI to modify the contents of the text control. However you can easily change it to enable the text control and try out this code without worrying about eval() causing you any harm.
Wrapping Up
In this chapter you learned several different approaches to creating a calculator using wxPython. You also learned a little bit about the pros and cons of using Python’s built-in eval() function. Finally, you learned that you can use Python’s ast and operator modules to create a finely-grained version of eval() that is safe for you to use. Of course, since you are controlling all input into eval(), you can also control the real version quite easily though your UI that you generate with wxPython.
Take some time and play around with the examples in this article. There are many enhancements that could be made to make this application even better. When you find bugs or missing features, challenge yourself to try to fix or add them.
Download the Source
The source code for this article can be found on Github. This article is based on one of the chapters from my book, Creating GUI Applications with wxPython.
Pingback: Mike Driscoll: Creating a Calculator with wxPython – Cebu Scripts
Pingback: Links 13/2/2019: Plasma 5.15.0 and a Look at Linux Mint Debian Edition Cindy | Techrights
Pingback: Creating a GUI Application for NASA’s API with wxPython | The Mouse Vs. The Python
Pingback: Mike Driscoll: Creating a GUI Application for NASA’s API with wxPython – Cebu Scripts